Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | ||||
4 | 5 | 6 | 7 | 8 | 9 | 10 |
11 | 12 | 13 | 14 | 15 | 16 | 17 |
18 | 19 | 20 | 21 | 22 | 23 | 24 |
25 | 26 | 27 | 28 | 29 | 30 | 31 |
Tags
- inheritance
- python
- 백준
- 객체지향
- 프림알고리즘
- 객체 지향
- 다형성
- nextInt
- 캡슐화
- 내부 클래스
- abstract
- 버퍼비우기
- 생성자
- Encapsulation
- Final
- java
- enum
- 추상화
- 제네릭
- 와일드카드
- 상속
- 추상 클래스
- 열거형
- 17472
- 인터페이스
- 최소신장트리
- Scanner
- this
- polymorphism
Archives
- Today
- Total
쫑쫑이의 블로그
백준 1766 문제집 Java [위상정렬, 우선순위 큐] 본문
https://www.acmicpc.net/problem/1766
1766번: 문제집
첫째 줄에 문제의 수 N(1 ≤ N ≤ 32,000)과 먼저 푸는 것이 좋은 문제에 대한 정보의 개수 M(1 ≤ M ≤ 100,000)이 주어진다. 둘째 줄부터 M개의 줄에 걸쳐 두 정수의 순서쌍 A,B가 빈칸을 사이에 두고 주
www.acmicpc.net
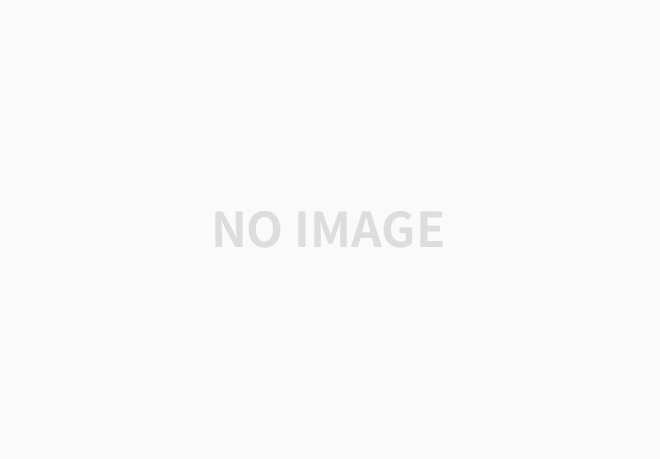
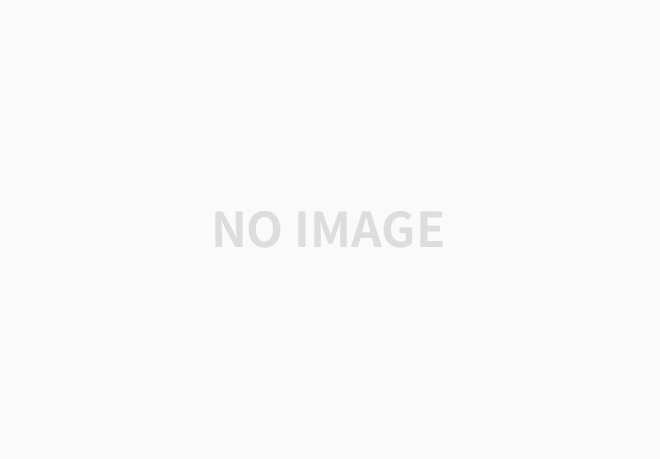
위상정렬과 우선순위큐를 사용해서 풀이하면 된다
입력 값에 맞게 가중치를 추가해주고
1부터 N까지 가중치가 0인 값만 우선순위큐에 넣어준 후
우선순위큐에서 하나씩 꺼내면서 출력값에 추가하고
꺼낸 값이 방문할 수 있는 문제 번호의 가중치를 감소하고
가중치가 0이 되면 우선순위큐에 넣는 과정을 반복한다
import java.io.*;
import java.util.*;
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st = new StringTokenizer(br.readLine());
StringBuilder sb = new StringBuilder();
int N = Integer.parseInt(st.nextToken());
int M = Integer.parseInt(st.nextToken());
PriorityQueue<Integer> queue = new PriorityQueue<>();
int[] degree = new int[N + 1];
ArrayList<Integer>[] next = new ArrayList[N + 1];
for (int i = 1; i <= N; i++) next[i] = new ArrayList<>();
for (int m = 0; m < M; m++) {
st = new StringTokenizer(br.readLine());
int A = Integer.parseInt(st.nextToken());
int B = Integer.parseInt(st.nextToken());
degree[B]++;
next[A].add(B);
}
for (int i = 1; i <= N; i++) {
if (degree[i] == 0) queue.add(i);
}
while (!queue.isEmpty()) {
int now = queue.poll();
sb.append(now).append(" ");
for (int n : next[now]) {
degree[n]--;
if (degree[n] == 0) queue.add(n);
}
}
sb.setLength(sb.length() - 1);
System.out.println(sb);
}
}
'알고리즘 > 백준' 카테고리의 다른 글
백준 2568 전깃줄 - 2 Java [LIS] (0) | 2022.11.11 |
---|---|
백준 4386 별자리 만들기 Java [최소스패닝트리, 프림] (0) | 2022.11.10 |
백준 1005 ACM Craft Java [위상정렬] (0) | 2022.11.07 |
백준 2623 음악프로그램 Java [위상정렬] (0) | 2022.11.06 |
백준 1647 도시 분할 계획 Java [최소스패닝트리, 프림] (0) | 2022.10.27 |